PHP语言入门教程
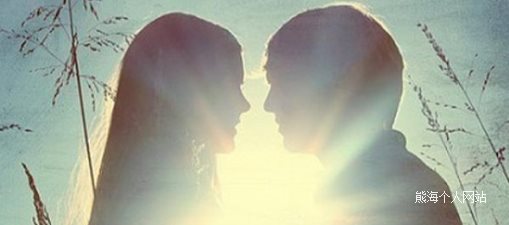
PHP 是一种广泛使用的开源脚本语言,并且是 Web 开发的重要工具之一。在本文中,我将为大家介绍 PHP 语言的基础知识,包括语法结构、变量、数据类型、函数、面向对象编程等方面的内容。
一、语法结构
PHP 的语法结构类似于 C 语言,使用花括号表示代码块。在 PHP 中,每条语句以分号结尾。
```php
if ($x > $y) {
echo "x is greater than y";
} else {
echo "y is greater than x";
}
```
二、变量
在 PHP 中,变量的名称必须以美元符号 $ 开头,后跟字母或下划线和字母的任意组合。变量的值可以是任意类型的数据。
```php
$name = "John";
$age = 25;
$height = 1.75;
$isMale = true;
```
三、数据类型
PHP 支持多种数据类型,包括标量类型(字符串、整数、浮点数、布尔值)、复合类型(数组、对象)和特殊类型(NULL、资源)等。
```php
// 字符串
$str = "Hello, World!";
// 整数
$int = 123;
// 浮点数
$float = 3.14;
// 布尔值
$bool = true;
// 数组
$arr = array(1, 2, 3);
// 对象
class Person {
public $name;
public $age;
}
$person = new Person();
$person->name = "John";
$person->age = 25;
// NULL
$nullVar = null;
// 资源
$file = fopen("myfile.txt", "r");
```
四、函数
在 PHP 中,可以使用 function 关键字来定义函数。函数的参数和返回值都需要显式声明类型。
```php
function add($x, $y) : int {
return $x + $y;
}
```
五、面向对象编程
PHP 支持面向对象编程。可以使用 class 关键字来定义类,使用 new 关键字来创建实例。
```php
class Person {
public $name;
public $age;
function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
function introduce() {
echo "My name is $this->name and I am $this->age years old.";
}
}
$person = new Person("John", 25);
$person->introduce();